How to inject enum object of java in spring framework?
Spring framework is a popular open-source framework developing applications in java. Enum
is a java enumeration keyword introduced in java 5 features.
In the Spring configuration file, we will inject different custom classes as well as predefined classes like Integer, String.
How to Inject Enum in Spring IOC container?
But injecting enum class in the spring container is different as if we do not correctly inject, we will end off with exceptions like “org.spring framework.beans.TypeMismatchException: Failed to convert property value of type”. so what is the solution of avoiding TypeMismatchException in spring?.
The solution is to use either custom property editors in spring custom configuration files we have to convert the enum to string to inject enum to class in spring.
This tutorial is to find out the ways to inject enum objects in spring. here is the enum definition java class
package com.cloudhadoop.constants;
enum Day {
MONDAY(0), TUESDAY(1), WEDNESDAY(2), THURSDAY(3), FRIDAY(4), SATURDAY(5), SUNDAY(
6);
private final int dayNumber;
private Day(int dayNumber) {
this.dayNumber = dayNumber;
}
public int getDayNumber() {
return dayNumber;
}
}
There are many ways to inject the enum object in the spring framework.
one way, is to inject the enum single value using enum Property as described in the below screenshot
<bean id="first" class="First">
<property name="weekday" value="1" />
</bean>
And the Java class for injection
class First{
private String weekday;
public void setWeekday(Integer weekday) {
this.weekday = Day.valueOf(weekday);
}
}
spring property editors are internally assigned the correct value to enum property
the second approach is to inject using factory-method as in the below screenshot.
The above approach is lightweight and the spring container validates the configuration when the container is started.
or another approach is to as is to assign all enum values using util: constant tag
<util:list id="days" value-type="com.cloudhadoop.constants">
<value>MONDAY</value>
<value>TUESDAY</value>
<value>WEDNESDAY</value>
<value>THURSDAY</value>
<value>FRIDAY</value>
</util:list>
Here is a spring component code example
import com.cloudhadoop.constants.Day;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class DayTest {
@Autowired
public Day day;
}
This approach provides for bean validation in IDE’s at development time. Please click the below screenshot for the spring code configuration file.
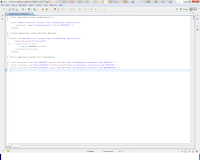
The three approaches work with enum in java with the spring 2.5.6 version.
Please share your comments on these topics.